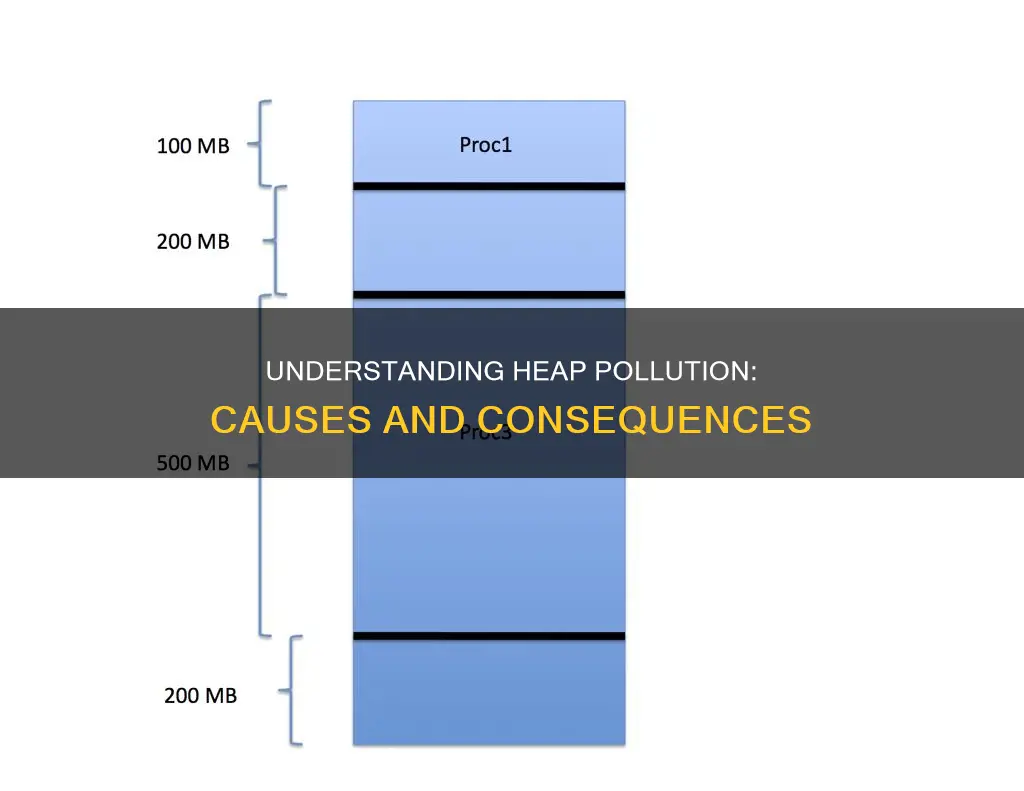
Heap pollution is a situation that occurs in Java programming when a variable of a parameterized type refers to an object that is not of that parameterized type. This results in bad data in the heap memory, where dynamic allocations are stored. Heap pollution can occur when type arguments and variables are not reified at run-time, leading to different parameterized types being implemented by the same class or interface. It is often detected during compilation and indicated with an unchecked warning, and can cause a ClassCastException during runtime. While heap pollution cannot be prevented, certain rules can be followed to avoid it, such as not using varargs parameters with generic types.
Characteristics | Values |
---|---|
Definition | Heap pollution is a situation that arises when a variable of a parameterized type refers to an object that is not of that parameterized type |
Cause | Mixing raw types and parameterized types, or performing unchecked casts |
Detection | Detected during compilation and indicated with an unchecked warning |
Runtime | Will often cause a ClassCastException |
Memory | Heap pollution implies that there is bad data in the heap memory |
Varargs | The use of varargs parameters with generic types can cause heap pollution |
What You'll Learn
Mixing raw types and parameterised types
Heap pollution in Java occurs when a variable of a parameterized type refers to an object that is not of that parameterized type. Mixing raw types and parameterised types can cause heap pollution.
A raw type is the name of a generic class or interface without any type arguments. For example, given the generic Box class:
> public class Box<T> {
> public void set(T t) { /* ... */ }
> }
To create a parameterized type of Box<T>, you supply an actual type argument for the formal type parameter T. Therefore, Box is the raw type of the generic type Box<T>. However, a non-generic class or interface type is not a raw type.
When mixing raw types and parameterised types, the compiler issues an unchecked warning to draw attention to potential heap pollution. This warning is generated if, either at compile-time or at runtime, the correctness of an operation involving a parameterised type cannot be verified. For example, when mixing raw types and parameterised types, or when performing unchecked casts.
> public void methodA() {
> List<String> parameterizedList = new ArrayList&<>();
> parameterizedList.add("Hello Folks");
> methodB(parameterizedList);
> }
>
> public void methodB(List rawList) {
> rawList.add(1);
> }
The code gets compiled (with a warning), and the Integer gets added to the raw type List when executed. The List<String> that was passed as an argument now contains a String and an Integer. The compiler prints out a warning due to the usage of raw types.
Toyota Batteries: Pollution or Clean Energy?
You may want to see also
Performing unchecked casts
Heap pollution is a critical concept in Java that often goes unnoticed until it leads to confusing runtime exceptions, specifically ClassCastException. It occurs when a variable of a parameterized type refers to an object that is not of that parameterized type. This situation is usually detected during compilation and indicated with an unchecked warning. Later, during runtime, heap pollution will often cause a ClassCastException.
Unchecked casts are a major cause of heap pollution. An unchecked warning is generated if, either at compile-time or at runtime, the correctness of an operation involving a parameterized type (for example, a cast or method call) cannot be verified. For example, heap pollution occurs when mixing raw types and parameterized types, or when performing unchecked casts. In normal situations, when all code is compiled at the same time, the compiler issues an unchecked warning to draw your attention to potential heap pollution. If you compile sections of your code separately, it is difficult to detect the potential risk of heap pollution.
Unchecked casts can introduce bugs if the actual type at runtime doesn't match the given type. For example, when working with Kotlin, you may encounter scenarios where type safety is compromised due to the use of an unchecked cast. This issue often arises when dealing with type casting in situations where the Kotlin compiler cannot verify the compatibility of types at compile time, leading to potential runtime exceptions.
To avoid the pitfalls of unchecked casts, it is recommended to use safe casting techniques like 'as?'' or 'filterIsInstance'. These techniques help to avoid runtime exceptions caused by invalid casts and handle nullable types seamlessly with fewer failures. Additionally, it is important to pay attention to warnings from the compiler, as they indicate potential issues. By using safe casting, you can avoid exceptions and handle invalid casts gracefully.
Understanding Heat Pollution: Causes and Sources Explained
You may want to see also
Using varargs parameters with generic types
Heap pollution in Java occurs when a variable of a parameterized type refers to an object that is not of that parameterized type. This situation is usually detected during compilation and indicated with an unchecked warning. Later, during runtime, heap pollution will often cause a ClassCastException.
Varargs, which were introduced in Java 5, provide a shorthand for methods that support an arbitrary number of parameters of one type. They help avoid writing boilerplate code by introducing a new syntax that can handle an arbitrary number of parameters automatically using an array. However, using varargs with generic types can lead to heap pollution.
When the compiler encounters a varargs method, it translates the varargs formal parameter into an array. However, Java does not permit the creation of arrays of parameterized types. As a result, the compiler converts the varargs formal parameter to an Object[] array, which can hold items of any type. This creates the possibility of heap pollution, as a value that does not match the parameterized type of the varargs formal parameter can be assigned to the Object[] array and, consequently, to the varargs formal parameter.
To prevent heap pollution, it is recommended to avoid using varargs parameters with generic types. Additionally, it is important to not cast an Object array to an array of a generic type and to avoid exposing the varargs parameter or the generic array to any other method. By following these rules, the risk of heap pollution can be mitigated.
Java
Public class ArrayBuilder {
Public static
For (T x : elements) {
ListArg.add(x);
}
}
Public static void faultyMethod(List
Object[] objectArray = l; // Valid
ObjectArray [0] = Arrays.asList(42);
String s = l [0].get(0); // ClassCastException thrown here
}
}
In the above code, the `addToList` method takes a varargs parameter `elements` of type `T...`. This is a generic type, which means it can be of any type specified by the caller. The `faultyMethod` method takes a varargs parameter `l` of type `List
Cows and Pollution: What's the Real Damage?
You may want to see also
Casting an object array to a generic type array
In the Java programming language, heap pollution can occur when type arguments and variables are not reified at run-time. As a result, different parameterized types are implemented by the same class or interface at run time, and a variable of a parameterized type may refer to an object that is not of that type. This situation is typically detected during compilation and indicated with an unchecked warning.
Generic methods that include variable arguments (varargs) parameters can cause heap pollution. For example, consider the following code:
Java
Public class ArrayBuilder {
Public static
For (T x : elements) {
ListArg.add(x);
}
}
Public static void faultyMethod(List
Object[] objectArray = l; // Potential heap pollution
ObjectArray[0] = Arrays.asList(42);
String s = l[0].get(0); // ClassCastException thrown here
}
}
In the `faultyMethod` method, the `Object[] objectArray = l;` statement can potentially introduce heap pollution. This is because a value that does not match the parameterized type of the varargs parameter `l` can be assigned to the `objectArray` variable, which is then assigned to `l`. This violates type safety and can lead to a ClassCastException, as seen in the subsequent line `String s = l[0].get(0);`>.
To avoid heap pollution, it is recommended to follow certain rules, such as avoiding the use of varargs parameters with generic types or casting an object array to an array of a generic type. Additionally, it is important to ensure that code compiles without warnings, as this guarantees the absence of heap pollution.
Plastics' Air Pollution: Harmful Toxins and Emissions
You may want to see also
Not compiling code all at once
In the Java programming language, heap pollution occurs when a variable of a parameterized type refers to an object that is not of that parameterized type. This situation is usually detected during compilation and indicated with an unchecked warning. Later, during runtime, heap pollution will often cause a ClassCastException.
When all code is compiled at the same time, the compiler issues an unchecked warning to draw attention to potential heap pollution. However, if sections of the code are compiled separately, it becomes difficult to detect the potential risk of heap pollution. This is because the compiler will not issue the same warning when code sections are compiled separately. Therefore, not compiling code all at once can be a cause of heap pollution.
In Java, heap pollution can occur when type arguments and variables are not reified at runtime. As a result, different parameterized types are implemented by the same class or interface at runtime. All invocations of a given generic type declaration share a single runtime implementation, which can result in the possibility of heap pollution.
To prevent heap pollution, it is recommended to avoid using varargs parameters with generic types or casting an object array to an array of a generic type. It is also important to avoid exposing the varargs parameter or the generic array to any other method. While heap pollution cannot be completely prevented, following these rules can help to reduce the risk.
Additionally, the use of the @SafeVarargs annotation can suppress warnings about potential heap pollution. However, it is important to note that this annotation does not prevent heap pollution from occurring. Instead, it mandates that the compiler is stricter when compiling code that uses it.
The Main Culprit Behind Pollution and Its Causes
You may want to see also
Frequently asked questions
Heap pollution is a technical term used in Java programming. It occurs when a variable of a parameterized type refers to an object that is not of that parameterized type. This results in bad data in the heap memory.
Heap pollution can cause a ClassCastException during runtime. This is when an operation is performed on a generic interface, and it contains a different type than declared.
Heap pollution is usually detected during compilation and indicated with an unchecked warning. However, it can be challenging to detect if the code is compiled separately.
Heap pollution can be caused by using varargs parameters with generic types or casting an Object array to an array of a generic type. It can also occur when mixing raw types and parameterized types or when performing unchecked casts.
While heap pollution situations cannot be entirely prevented, certain rules can help reduce the risk. These include avoiding the use of varargs parameters with generic types and not exposing the varargs parameter or the generic array to any other method.